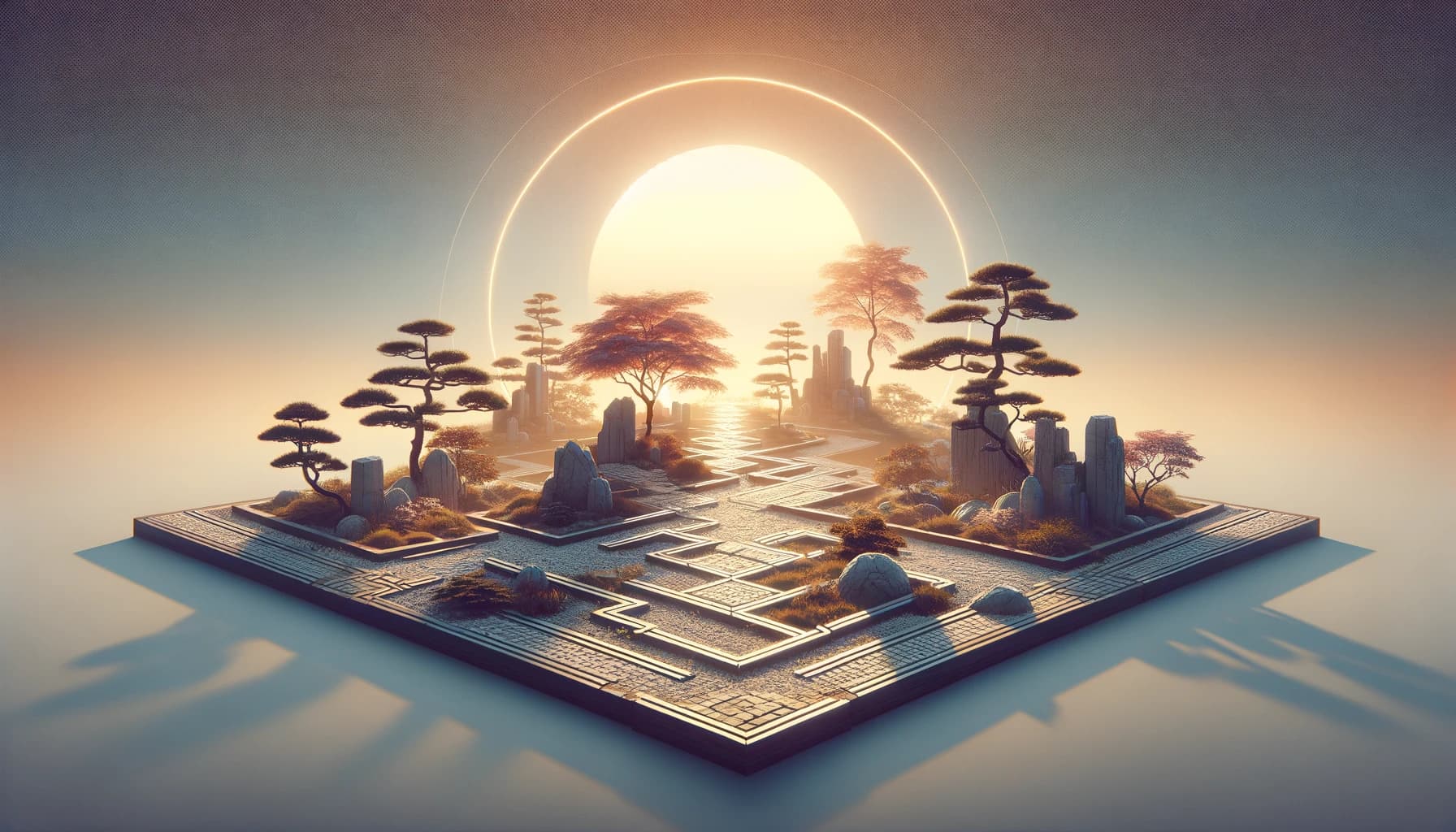
The Event Loop
The Zen Garden
Imagine a Zen garden, a place of tranquility and order, where a single gardener (the JavaScript engine) tends to the garden. The gardener follows a specific path (the event loop), picking up tasks (events or callbacks) from various parts of the garden (the call stack and the task queue). The gardener can only handle one task at a time, but they ensure that the garden remains a place of harmony by efficiently managing these tasks, allowing for a smooth flow of events and operations.
The Gardener's Path
Gardener treads paths,
Tasks gathered in silent stride,
Zen garden's calm flow.
The Zen Garden Event Loop
// The garden represents the event loop
function tendToGarden() {
console.log("Tending to the Zen garden"); // Immediate task
}
// High-tech tools represent asynchronous operations
setTimeout(() => {
console.log("Watering plants with a robotic arm"); // Asynchronous task
}, 1000);
console.log("Starting to tend the garden"); // Synchronous task
tendToGarden(); // Call the function to tend to the garden
console.log("Garden tending complete"); // Synchronous task continues
// Output:
// Starting to tend the garden
// Tending to the Zen garden
// Garden tending complete
// Watering plants with a robotic arm (after a delay, representing asynchronous execution)
Callback
"Gardener Tends Async"
G represents the Garden's continuous care, T stands for the Tasks managed, and A emphasizes the Asynchronous nature of the operations.
Concept Checking Questions
-
What is the role of the event loop in JavaScript?
- The event loop allows JavaScript to perform non-blocking, asynchronous operations by continuously monitoring the call stack and task queue, executing tasks when the stack is clear.
-
How does JavaScript handle asynchronous tasks?
- Asynchronous tasks are handed off to the Web APIs environment, allowing the call stack to continue running. Once these tasks are complete, they are moved to the task queue, where the event loop will eventually execute them.
-
What happens when the call stack is not empty?
- The event loop waits for the call stack to become empty before executing tasks from the task queue, ensuring a smooth, non-blocking flow of execution.