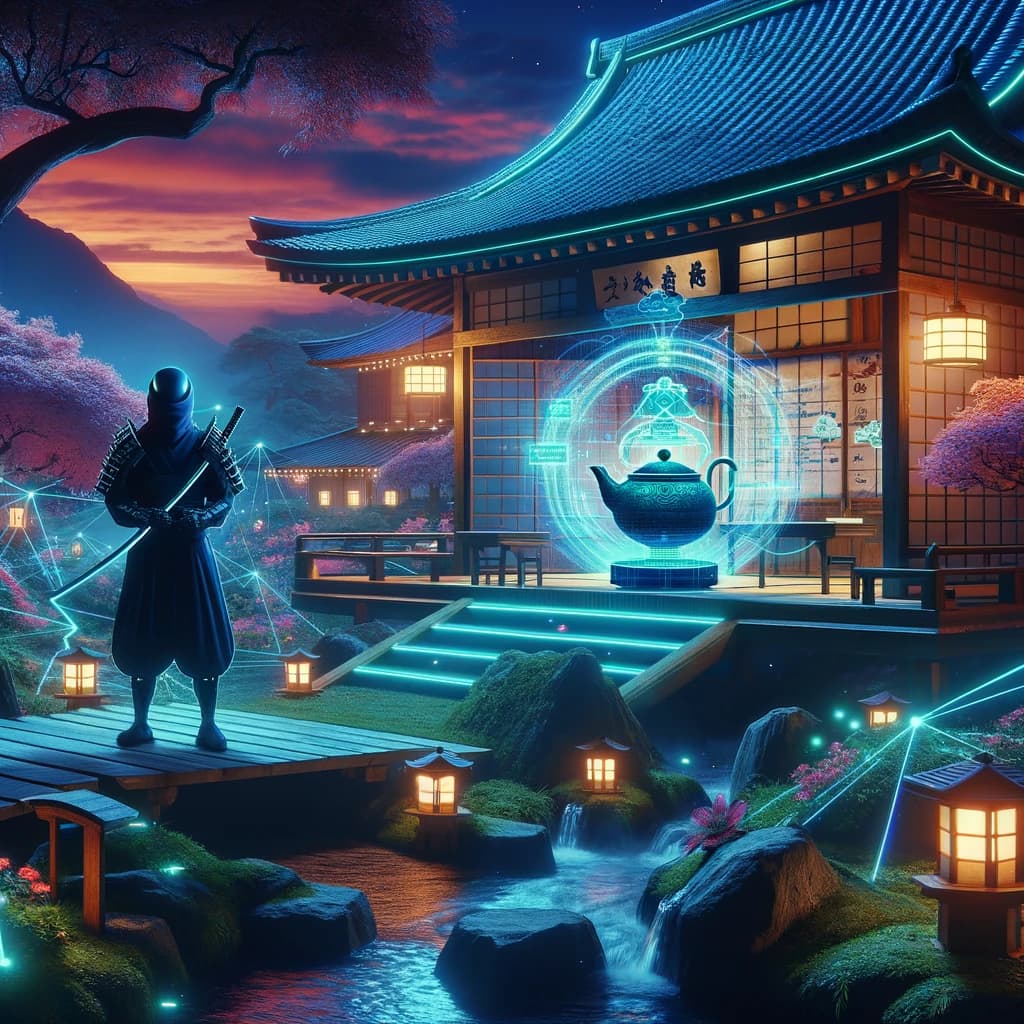
Promises
Written on by PadoSensei.
The Tea Ceremony
Think of a JavaScript promise like a traditional Japanese tea ceremony. The ceremony (promise) begins with a pledge to serve tea (resolve a value), but it takes time to prepare. The tea might be served perfectly (fulfilled promise), or there might be an issue, like running out of tea leaves (rejected promise), but you're assured of receiving a clear outcome.
The Promise Of Tea
Await in silence,
Brewing future, cup in hand,
Fulfill or spill—fate.
The Tea Ceremony Promise
// The Tea Ceremony analogy
const teaCeremony = new Promise((resolve, reject) => {
// Preparing the tea
let teaReady = true; // Change to false to see rejection
if(teaReady) {
resolve("Tea is served."); // Tea prepared successfully
} else {
reject("The ceremony cannot proceed."); // An issue occurred
}
});
// Attending the ceremony
teaCeremony.then((message) => {
console.log(`Success: ${message}`); // Handling fulfillment
}).catch((error) => {
console.log(`Error: ${error}`); // Handling rejection
});
Promise
Provisional Response Offering Meticulous Insight into Success or Error
Concept Checking Questions
1. What does the resolve
function represent in the tea ceremony analogy?
- Answer: The resolve function represents the successful preparation and serving of the tea, fulfilling the promise of the tea ceremony.
2. What happens if teaReady
is set to false
?
- Answer: If
teaReady
is set tofalse
, the promise is rejected, symbolizing the ceremony cannot proceed due to an issue, such as running out of tea leaves.
3. How do .then()
and .catch()
relate to the ceremony?
- Answer:
.then()
handles the scenario where the tea is served successfully (fulfillment), and.catch()
deals with any issues that prevent the tea from being served (rejection), maintaining the flow of the ceremony regardless of the outcome.