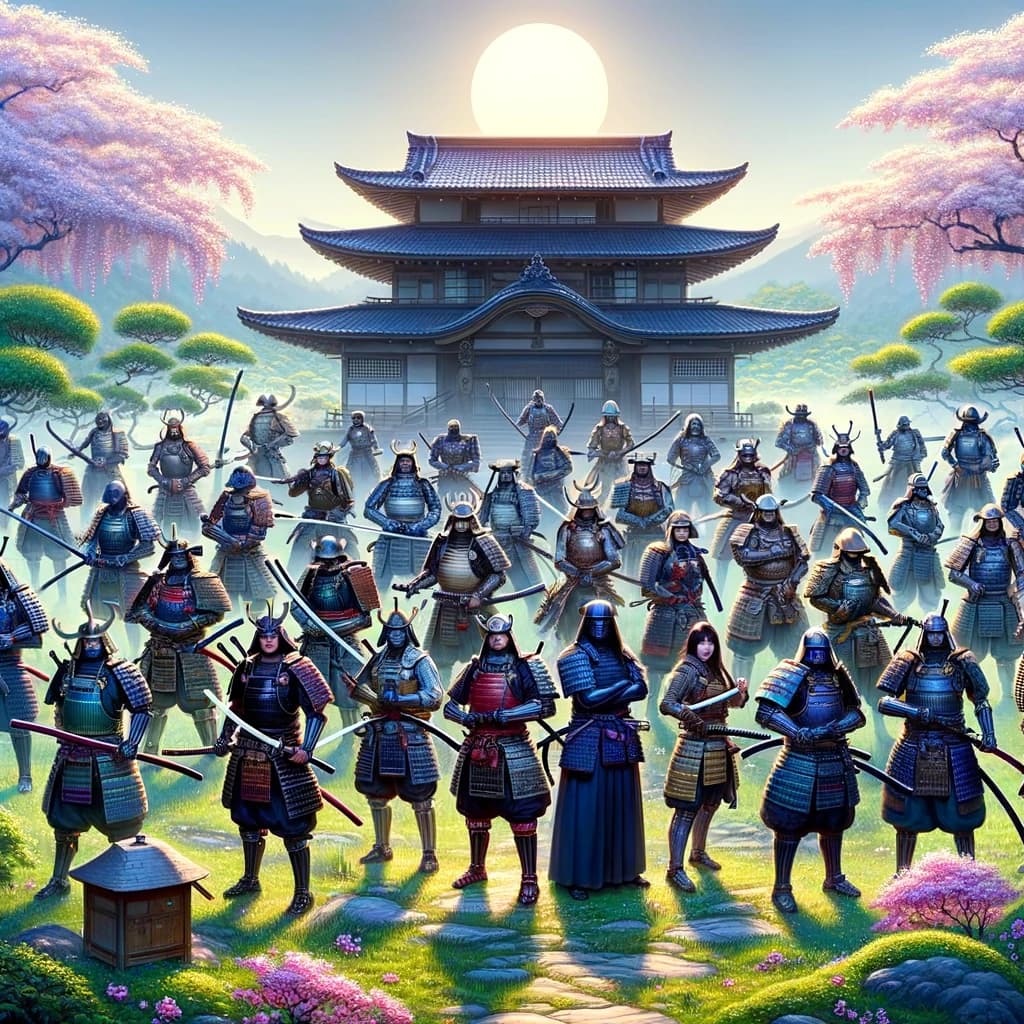
OOP in Javascript
The Samurai Clan
Imagine JavaScript's OOP as a Samurai Clan. Each samurai (object) has their own skills (methods) and attributes (properties). The clan's hierarchy (prototype chain) determines how skills and attributes are inherited, with the legendary ancestors (prototypes) passing down their revered techniques and traits to their descendants. The dojo (class) serves as the blueprint for training new samurai, ensuring each member shares a common foundation, yet allows for individual growth and specialization.
Echoes of Inheritance
Ancient dojo stands,
Samurai inherit strength,
Code weaves like swift streams.
The Samurai Clan
// Defining the dojo (class)
class Samurai {
constructor(name, rank) {
this.name = name;
this.rank = rank;
}
// A method akin to a skill
showHonor() {
console.log(`${this.name}, of rank ${this.rank}, shows honor.`);
}
}
// Creating an instance of a samurai
const samurai1 = new Samurai("Takashi", "Warrior");
// The legendary ancestor passing down techniques (using prototype)
Samurai.prototype.demonstrateSkill = function(skill) {
console.log(`${this.name} demonstrates ${skill}.`);
};
// Samurai demonstrating a skill
samurai1.showHonor(); // Takashi, of rank Warrior, shows honor.
samurai1.demonstrateSkill("the way of the sword"); // Takashi demonstrates the way of the sword.
Object Orientated Programming in Javascript
Classes And Prototypes Manage Objects: CAPMO
This helps remember that Classes and Prototypes are key to managing objects in JavaScript's approach to OOP.
Concept Checking Questions
-
What is the role of a class in JavaScript OOP?
- A class acts as a blueprint for creating objects, defining initial properties and methods.
-
How do prototypes contribute to OOP in JavaScript?
- Prototypes allow for property and method inheritance among objects, enabling shared behavior across instances.
-
Can you explain how methods in JavaScript objects are used?
- Methods are functions stored as object properties, used to define actions that an object can perform, often manipulating the object's own properties or interacting with other objects.