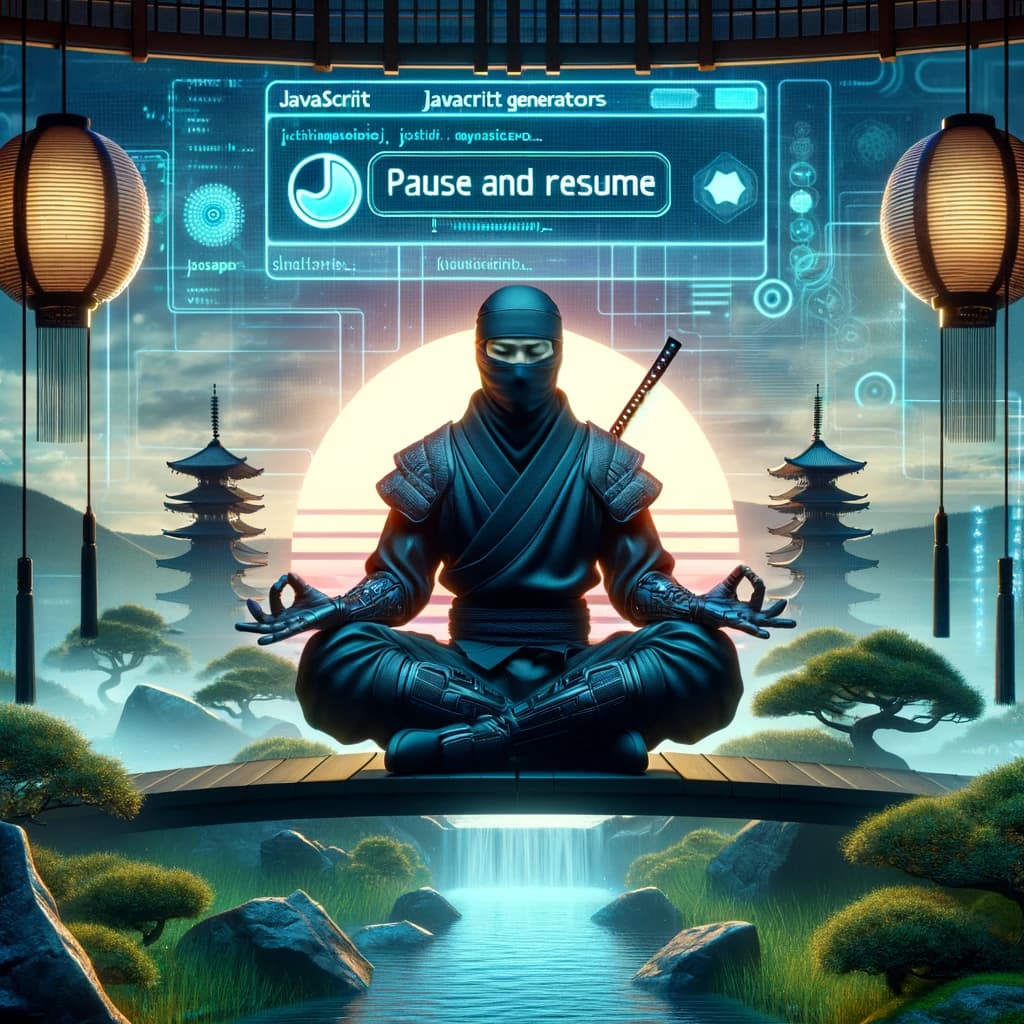
Generators
Yield in the Zen Garden
Imagine you're in a Japanese Zen garden, where a garden master is creating patterns in the sand. Instead of completing the entire garden in one go, the master methodically works on a small section, pauses to contemplate the next steps, and then resumes the work. This process of action, pause, and continuation is similar to how generators function in JavaScript.
Generative Flow
In the Zen garden,
Pausing steps, then moving on,
Generators flow.
Zen Garden Generator
function* zenGardenCreation() {
yield 'Rake sand'; // First step, raking the sand
yield 'Place stones'; // Placing stones carefully
yield 'Trim plants'; // Trimming the plants
// The garden master can pause and resume at each step
}
const gardenMaster = zenGardenCreation();
console.log(gardenMaster.next().value); // Rake sand
console.log(gardenMaster.next().value); // Place stones
console.log(gardenMaster.next().value); // Trim plants
Generators
Garden Pauses Work
"Garden Pauses Work," where "Garden" stands for Generators, "Pauses" reflects the ability to pause execution, and "Work" signifies the resumption of function execution.
Concept Checking Questions
-
What does the
yield
keyword do in a generator function?- Answer: The
yield
keyword pauses the generator function's execution and returns a value.
- Answer: The
-
Can a generator function run indefinitely?
- Answer: Yes, a generator function can run indefinitely if it's designed to loop endlessly or wait for new inputs.
-
How do you resume the execution of a paused generator function?
- Answer: You resume the execution by calling the
next()
method on the generator object.
- Answer: You resume the execution by calling the